Unveiling the Mystery: Choosing the Best Metric for Tracking Test Coverage in Laravel with PHPUnit
Let's delve deeper into the world of test coverage in Laravel projects using PHPUnit. You've got three key metrics at your disposal: Classes, Methods, and Lines. Each metric offers a unique lens through which to view your testing efforts, and your recent team retreat sparked a discussion favoring Methods. Before solidifying this choice, let's explore the strengths and weaknesses of each metric with examples to guide you towards the most effective approach for tracking progress.
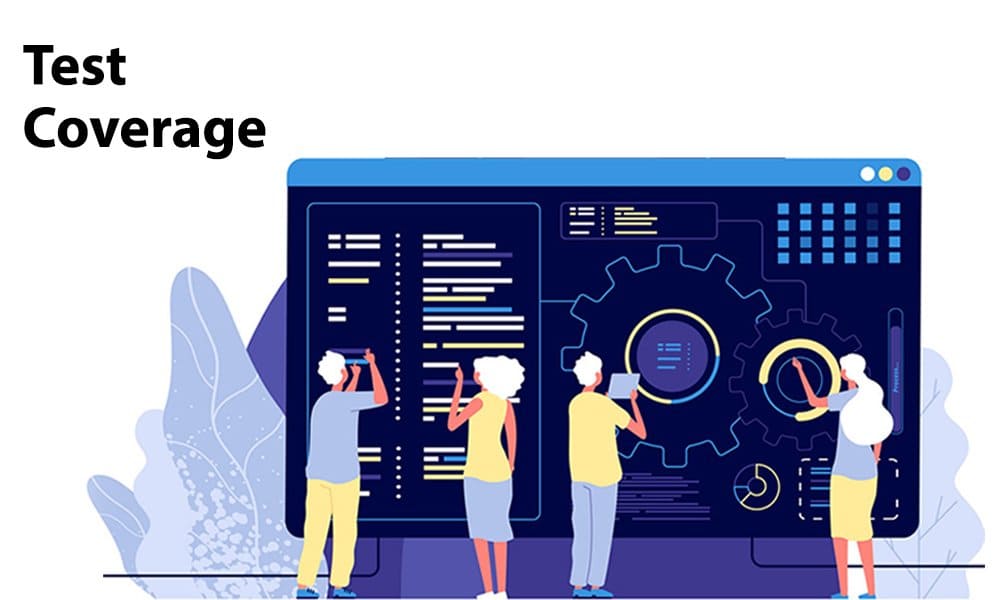
Demystifying the Metrics with Examples:
-
Classes: Imagine this metric as a headcount for your test suite. It reveals the percentage of classes that have at least one test case associated with them. Here's an example:
- You have a
User
class with functionalities like registration, login, and profile updates. - Class Coverage: 100% (because you have at least one test for
User
).
This doesn't tell you if the test actually verifies all functionalities of the
User
class. It could just be a basic test for registration. - You have a
-
Methods: This metric gets more granular, focusing on the percentage of methods within your classes that have test coverage. Let's see how it impacts the example:
- You have a
registerUser
method within theUser
class. - Method Coverage: 50% (because you only have a test for a happy path registration scenario).
This indicates you might be missing edge cases like invalid email registration or empty password scenarios.
- You have a
-
Lines: This metric goes line by line, calculating the percentage of individual lines of code that are covered by tests. Here's why it can be misleading:
- Imagine a
registerUser
method with 10 lines of code, but 2 lines are comments explaining the logic. - Lines Coverage: 80% (because 8 out of 10 lines are covered by the test).
This doesn't necessarily mean the core functionality of registration is thoroughly tested. The comments themselves don't require testing.
- Imagine a
Why Methods Might Not Be the Silver Bullet:
While Methods offer a seemingly balanced approach, here's why it might not be the perfect choice:
- Incomplete Method Coverage: Just because a method has a test doesn't guarantee it's a good test. The test might only cover a specific scenario, leaving other functionalities untested.
- Quantity Over Quality Trap: Chasing a high Methods coverage percentage can lead to writing numerous shallow tests that don't truly validate the intended behavior.
The Path to Effective Tracking: A Multi-Metric Approach
Instead of relying solely on Methods, consider a more strategic approach that combines different metrics:
- Set a Minimum Threshold for Class Coverage: This ensures a baseline level of testing across your entire codebase. Every class should have at least one test, but strive to write comprehensive tests that cover all functionalities.
- Track Method Coverage as a Secondary Metric: Monitor Method coverage alongside Class coverage. Aim for a steady increase, but prioritize writing high-quality tests that comprehensively cover the logic within each method.
- Monitor Lines Coverage for Information Only: Keep an eye on Lines coverage, but don't make it your primary goal. It can be a helpful indicator, but don't get caught up in achieving a specific percentage.
Generating Your Test Coverage Report:
PHPUnit provides built-in functionality to generate test coverage reports. These reports offer a detailed breakdown of how much of your codebase is covered by tests, measured in terms of Classes, Methods, and Lines. Here's how to generate a report:
-
Navigate to your project directory: Open your terminal and use the
cd
command to navigate to the root directory of your Laravel project. -
Execute the PHPUnit Command: Run the following command, replacing
<path/to/tests>
with the actual path to your test files and<coverage-output-directory>
with the desired location for the report:This command instructs PHPUnit to generate a text-based coverage report and store it in the specified directory.
-
Open the Coverage Report: Navigate to the directory you specified in the
<coverage-output-directory>
and open the generated file (usually namedindex.html
). This will display a detailed report with coverage information for your classes, methods, and lines of code.
Additional Tips for Boosting Your Test Coverage Journey:
- Prioritize Critical Functionalities: Focus your testing efforts on the core functionalities of your application and areas that are more prone to errors. This ensures your tests provide the most value.
- Regular Code Coverage Report Reviews: Schedule regular reviews