Behind the Scenes of a Laravel Application: The Power of Jobs and Queues
Laravel, a robust PHP framework, empowers developers to craft dynamic web applications. One of its hidden gems, the queuing system, plays a crucial role in maintaining smooth operation and exceptional user experiences. By leveraging jobs and queues, you can offload time-consuming tasks, ensuring your application remains responsive and efficient under heavy workloads.
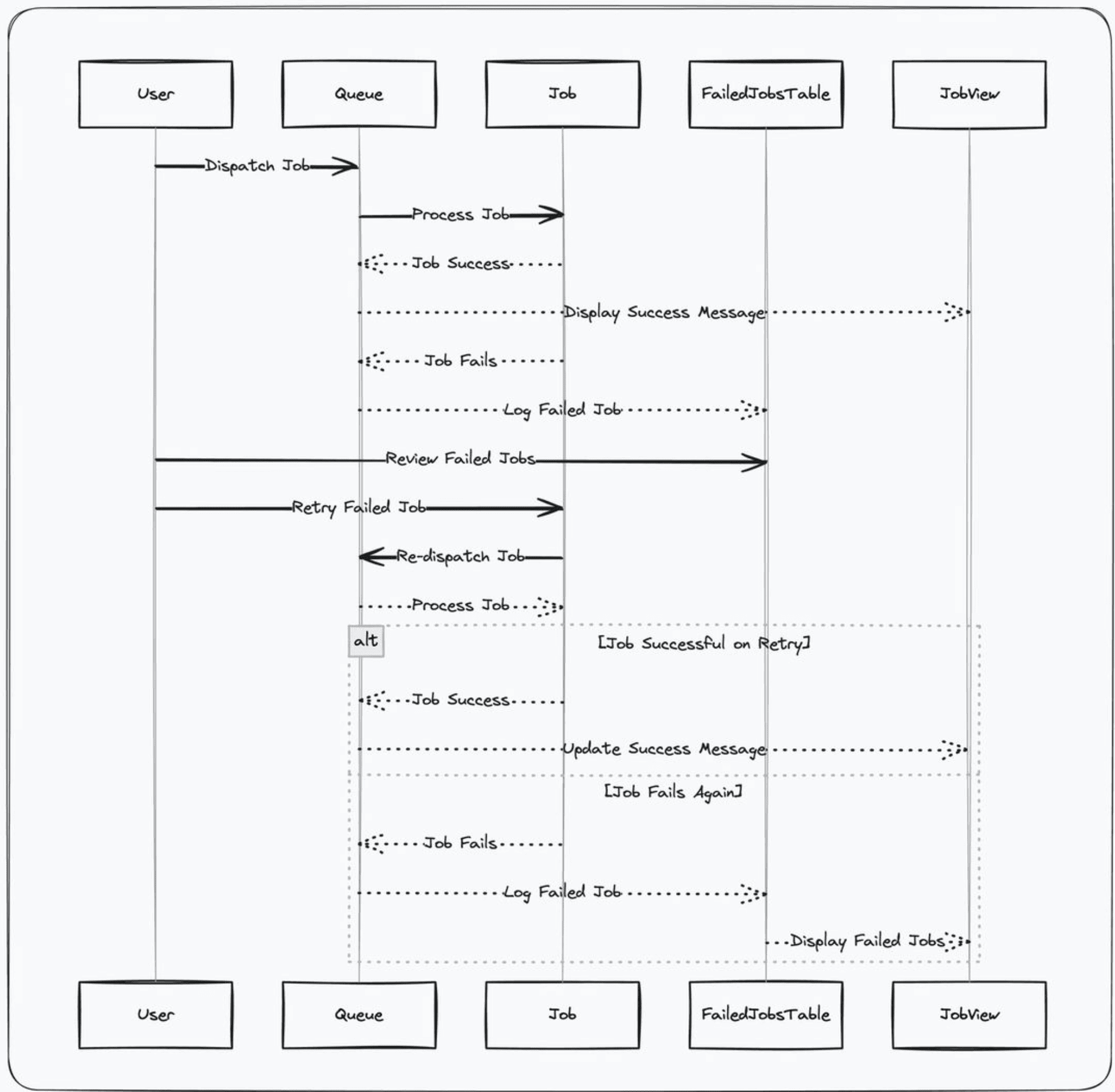
Understanding the Players: Jobs and Queues
-
Jobs: Imagine these as individual work units that can be queued and processed asynchronously. They function independently of the main application flow, allowing background execution without hindering user interaction. Laravel provides a convenient
make:job
Artisan command to generate job classes. -
Queues: Think of them as organized waiting lines for jobs. When a job is dispatched, it's added to a specific queue. Worker processes, acting as dedicated attendants, then retrieve and process jobs one by one from these queues.
The Seamless Collaboration: How Jobs and Queues Work Together
-
Initiating the Process: A user action triggers an event in your Laravel application. This could be anything from submitting a form to uploading a large file.
-
Dispatching the Job: The application dispatches a job to the designated queue. This job encapsulates the specific task instructions, including any necessary data or objects. You can use the
dispatch
method on the job class to achieve this. -
Worker Takes the Stage: A worker process, constantly monitoring the queue, retrieves the job at the front of the line. It then proceeds to execute the defined logic within the job's
handle
method. -
Job Completion and Beyond: Once the job finishes its task, the worker might perform follow-up actions like updating the database, sending email notifications, or logging completion messages.
Benefits of Utilizing Jobs and Queues
Integrating jobs and queues into your Laravel application unlocks a plethora of advantages:
- Enhanced Performance: By delegating long-running or resource-intensive tasks to the background, your application maintains responsiveness for your users. They won't experience delays while the application processes these tasks.
- Superior Scalability: Queues empower you to scale your application horizontally. Simply add more worker processes to handle increased job volumes and traffic surges. This ensures smooth operation even under heavy load.
- Elevated User Experience: Users no longer have to wait for lengthy tasks to finish before interacting with other parts of your application. This translates to a more fluid and enjoyable user experience.
Code Example: Processing Uploads Asynchronously
Let's delve into a practical example showcasing how jobs and queues can be harnessed in a Laravel application. Imagine an e-commerce platform where users upload product images. Here's how we can leverage this powerful duo:
In this scenario:
- We create a
ProcessProductImage
job class using themake:job
Artisan command. - The constructor takes the uploaded image and product ID as arguments.
- The
handle
method resizes the image, saves it to storage, and updates the product record in the database with the resized image path. - In your controller, when handling the product image upload, you can dispatch this job:
Here's a breakdown of the changes:
- We first create the product record in the database using
Product::create
. - The uploaded image is stored in a temporary location using the
store
method of the uploaded file instance. - We then dispatch the
ProcessProductImage
job using thedispatch
method. We pass the temporary image path and the newly created product's ID as arguments. - Finally, the user is redirected to the product listing page with a success message.
By dispatching the image processing job, we ensure that the user doesn't experience any delay while the image is being resized and saved. The application can continue serving other requests while the worker process takes care of the image processing task in the background.
Configuring Queues and Workers
Out of the box, Laravel supports various queue drivers like database, Redis, and Beanstalkd. The optimal choice depends on your application's specific needs and infrastructure. Configuring queues is straightforward; you can define the driver, connection settings, and worker settings within the config/queue.php
configuration file.
Worker processes are the backbone of queue functionality. They are responsible for continuously fetching and processing jobs from the designated queues. Laravel provides a php artisan queue:work
command to start a worker process. Additionally, for production environments, you can utilize tools like Supervisor or Laravel Horizon to manage and monitor worker processes for optimal performance.
Handling Job Failures and Retries
Even in the most robust systems, unexpected errors can occur. Queues provide mechanisms to gracefully handle job failures. Laravel allows you to configure the number of times a failed job should be retried before being marked as permanently failed. Additionally, you can define custom logic for handling these failed jobs, such as sending notifications or logging detailed error messages for debugging purposes.
Advanced Queuing Features
Laravel offers a rich set of features to further enhance your queuing experience:
- Delayed Jobs: Schedule jobs for execution at a specific time in the future.
- Chained Jobs: Execute a sequence of jobs one after another, ensuring a specific order of operations.
- Exclusive Jobs: Prevent multiple workers from processing the same job simultaneously, useful for critical tasks.
Conclusion: A Well-Oiled Machine
By leveraging jobs and queues effectively, you can transform your Laravel application into a well-oiled machine. Improved performance, exceptional scalability, and a seamless user experience are just some of the rewards you reap. Explore the various queue drivers, delve deeper into advanced queuing features, and watch your application thrive under even the most demanding workloads.
Remember, a well-designed queueing system is a valuable asset for any Laravel application. It allows you to focus on core functionalities while ensuring smooth background operations for a truly exceptional user experience. So, the next time you're building a Laravel application, don't underestimate the power of jobs and queues – they might just be the secret ingredient your application needs for long-lasting success.