Laravel Pint is a highly efficient tool designed to streamline code formatting and ensure adherence to coding standards in Laravel projects. By incorporating Pint into your development workflow, you can maintain a consistent code style, minimize errors, and enhance readability across your team.
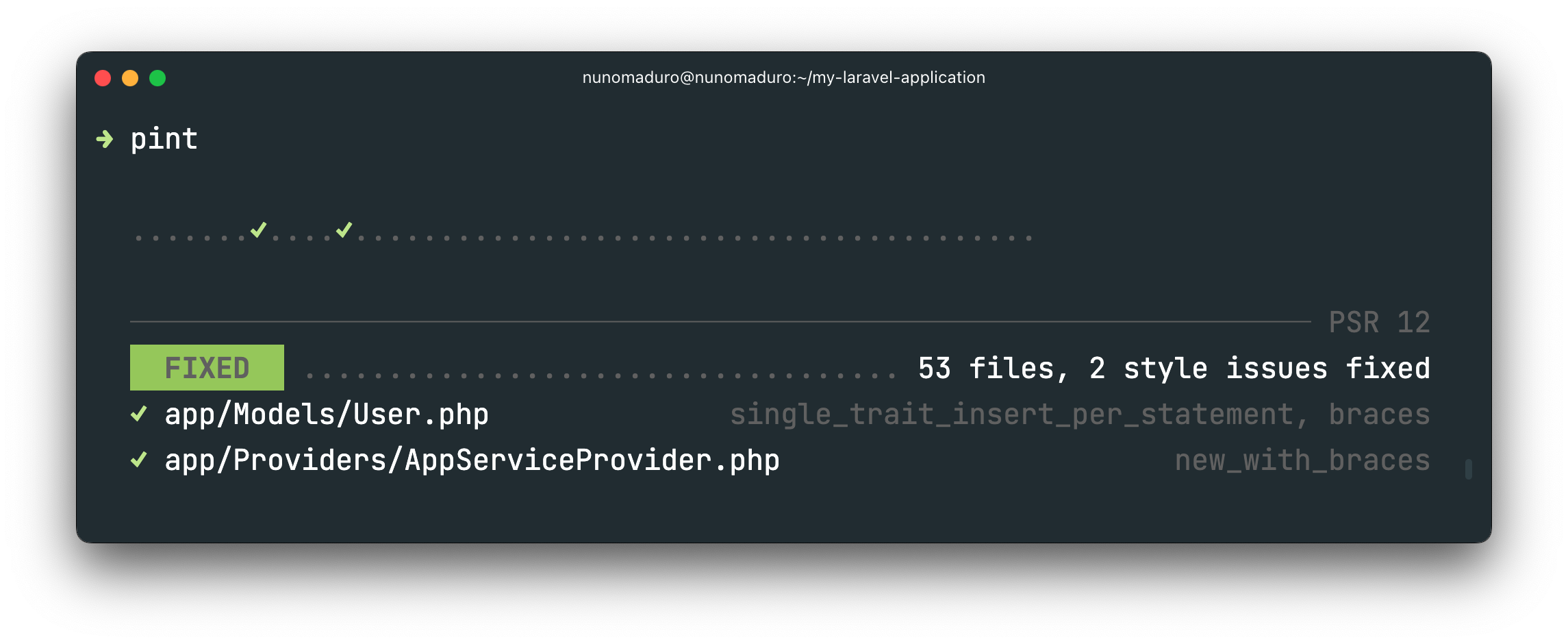
What is Laravel Pint?
Laravel Pint is an opinionated code formatting tool built on top of PHP CS Fixer. It's designed to work seamlessly with Laravel, helping you enforce coding standards and best practices with minimal configuration. Pint aims to reduce friction in code reviews and eliminate the need for manual formatting, allowing you to focus on writing clean and maintainable code.
Why Use Laravel Pint?
- Consistent Code Style: Ensures a uniform code style throughout the project, making it easier to read and maintain.
- Automated Formatting: Automatically formats code based on predefined rules, reducing the time spent on manual adjustments.
- Improved Code Quality: Enforces best practices and coding standards, minimizing common coding errors.
Installing Laravel Pint
To install Laravel Pint, you can use Composer. Run the following command in your terminal:
Once installed, you can run Pint using:
This will scan your project and automatically fix any code style issues it finds.
Default Configuration of Laravel Pint
Laravel Pint comes with a default configuration that follows Laravel’s coding standards. Here’s a detailed explanation of the preset rules used by Pint:
Array Formatting
array_indentation
: Enforces indentation for multi-line arrays.array_syntax
: Uses the short array syntax ([]
) instead of the long syntax (array()
).
Binary Operators
binary_operator_spaces
: Ensures a single space around binary operators for readability.
Blank Lines
blank_line_after_namespace
: Inserts a blank line after the namespace declaration.blank_line_after_opening_tag
: Adds a blank line after the PHP opening tag.blank_line_before_statement
: Adds a blank line beforecontinue
andreturn
statements.blank_line_between_import_groups
: Inserts a blank line between import groups.blank_lines_before_namespace
: Adds blank lines before the namespace declaration.
Braces Position
braces_position
: Defines the position of braces for various code structures:- Control structures (like
if
,for
, etc.) keep the opening brace on the same line. - Function and class declarations have the opening brace on the next line unless the signature is on a new line.
- Control structures (like
Casting and Constants
cast_spaces
: Ensures a single space around type casts.constant_case
: Converts constants to lower case (true
,false
,null
).
Class and Function Definitions
class_attributes_separation
: Requires separation between class attributes (const
,method
,property
).class_definition
: Allows single-line class definitions if possible.
Code Clarity
clean_namespace
: Removes unused namespaces.compact_nullable_type_declaration
: Uses compact syntax for nullable type declarations.concat_space
: No spaces around concatenation operator.fully_qualified_strict_types
: Not enforced, allows flexible type usage.function_declaration
: Enforces standard function declaration format.
Control Structures
control_structure_braces
: Requires braces for control structures.elseif
: Enforces the use ofelseif
overelse if
.control_structure_continuation_position
: Keepselse
,elseif
,catch
, etc., on the same line as the closing brace of the previous control structure.
Whitespace and Line Breaks
indentation_type
: Uses spaces for indentation.line_ending
: Enforces Unix-style line endings (\n
).single_blank_line_at_eof
: Adds a blank line at the end of files.no_trailing_whitespace
: Removes trailing whitespace.
PHPDoc and Comments
phpdoc_order
: Orders PHPDoc tags (param
,return
,throws
) for consistency.phpdoc_align
: Aligns PHPDoc annotations for readability.phpdoc_summary
: Omits a summary in PHPDoc comments.
Other Formatting Rules
method_chaining_indentation
: Enforces indentation for method chains.no_unused_imports
: Removes unused import statements.ordered_imports
: Orders imports alphabetically and by type (const, class, function).single_quote
: Enforces the use of single quotes for strings.visibility_required
: Requires visibility to be declared for methods and properties.
Customizing Pint Configuration
While the default configuration is well-suited for most Laravel projects, you might want to customize it to fit your specific needs. You can do this by creating a .pint.json
file in the root of your project and defining your custom rules. Here’s an example:
Conclusion
Laravel Pint is a powerful tool for maintaining a clean and consistent codebase. By automating code formatting and enforcing Laravel's coding standards, it helps you focus on building robust applications without worrying about code style inconsistencies.
Integrating Laravel Pint into your development workflow not only saves time but also promotes best practices, leading to more maintainable and readable code. Whether you're working on a small project or a large enterprise application, Laravel Pint ensures that your code remains in top shape.
For more details and advanced usage, check out the official Laravel Pint documentation.
Feel free to share this blog with your team or fellow developers to help them understand and implement Laravel Pint in their projects!